Android Integration
Configure FCM
Firebase Cloud Messaging is a service that allows you to send notifications to your applications. If you don’t have your own Firebase integration, Then Goto FCM (https://console.firebase.google.com/) and register your app. Copy the Sender-Id and Legacy server key from Fcm. Download the latest config JSON file (google-service.json ) and paste it into your app folder (my-app/android/app).
Note:- In the Case of Cordova Integration. Keep this google-service.json file in your project root folder.
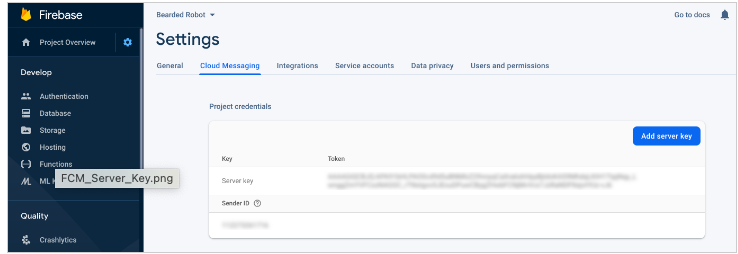
Copy Sender ID and Legacy Server Key
Goto NotifyVisitors panel and from the App push menu go to Android inside the configuration section from the left side menu panel and Paste the Sender-Id and Legacy server key.
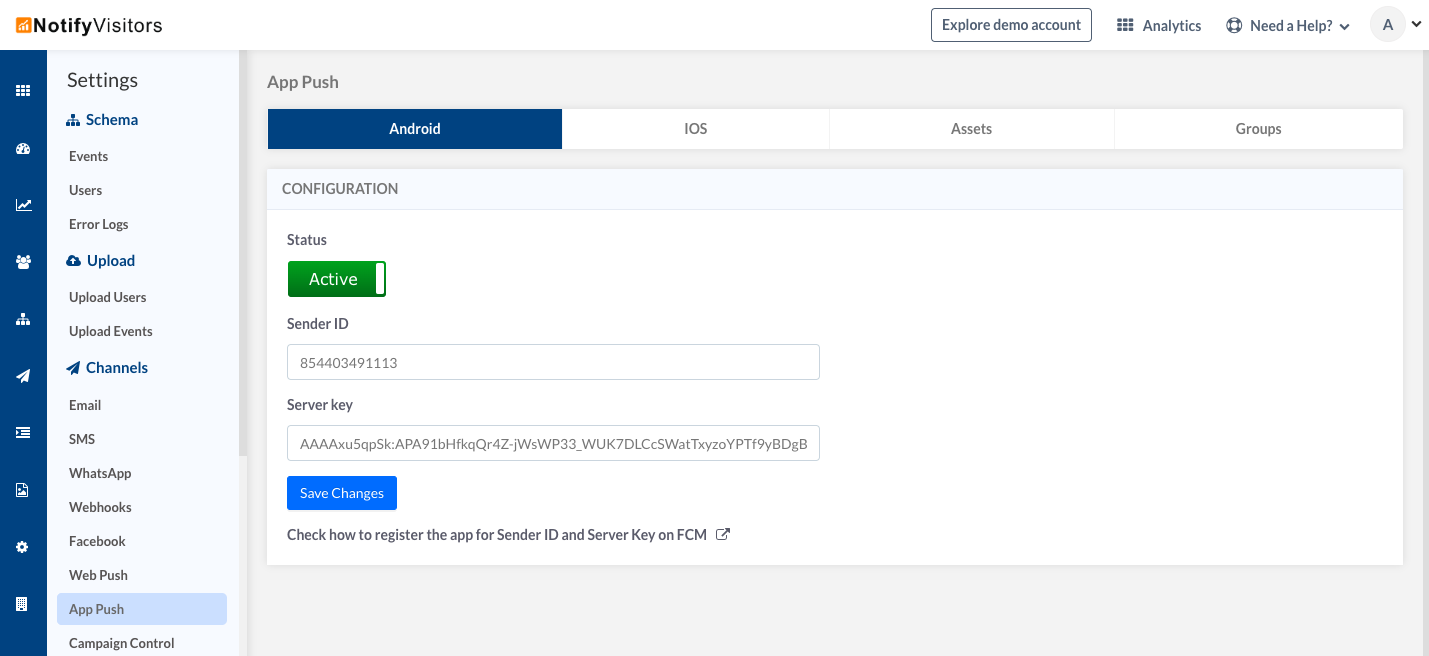
Configure Sender ID and Legacy-Server-Key on NotifyVisitors Panel
Push Notification Icon
Create a monochrome png icon and the size must be 200×200. The name of the icon should be sm_push_logo.png Paste into your-project/android/app/src/main/res/drawable/.
Note:- In the Case of Cordova Integration. Keep this icon in your project root folder
Configure AndroidManifest.xml
<manifest….>
<uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW"/>
<uses-permission android:name="android.permission.WAKE_LOCK"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<uses-permission android:name="android.permission.CHANGE_NETWORK_STATE"/>
<uses-permission android:name="android.permission.CHANGE_WIFI_STATE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application>
<meta-data
android:name="notifyvisitors_bid"
android:value="5499" />
<meta-data
android:name="notifyvisitors_bid_e"
android:value="DA85CA5D75F7920320C8244AF91D448A"/>
</application>
/* Open the app Config.xml file and paste the below code */
/* Under Android Platform Section */
<config-file parent="application" target="AndroidManifest.xml">
<meta-data android:name="notifyvisitors_bid" android:value="5499" />
<meta-data android:name="notifyvisitors_bid_e" android:value="DAXXXXXXXXXXXXXXXXXXXXXXXXX" />
</config-file>
/* Under IOS Platform Section */
<config-file parent="nvBrandID" target="*-Info.plist">
<string>54XX</string>
</config-file>
<config-file parent="nvSecretKey" target="*-Info.plist">
<string>DA85XXXXXXXXXXXXXXXXXXXXXXXXX8</string>
</config-file>
Note:
In the above example, Dummy Brand ID and Encryption keys are shown. Kindly login to your Notifyvisitors account to see your credentials.
Initialize SDK
A. If you have an Application class Otherwise skip this step.
Import below package
import com.rn_notifyvisitors.RNNotifyvisitorsModule;
import com.flutter.notifyvisitors.NotifyvisitorsPlugin;
import com.notifyvisitors.notifyvisitors.NotifyVisitorsApplication;
Call the above method in onCreate()
RNNotifyvisitorsModule.register(this);
//Example
public void onCreate() {
super.onCreate();
RNNotifyvisitorsModule.register(this);
SoLoader.init(this, /* native exopackage */ false);
}
NotifyvisitorsPlugin.register(this);
//Example
public void onCreate() {
super.onCreate();
NotifyvisitorsPlugin.register(this);
}
NotifyVisitorsApplication.register(this);
//Example
public void onCreate() {
super.onCreate();
NotifyvisitorsPlugin.register(this);
}
B. If you don't have an Application class. Then add the below line to your app manifest.xml.
android:name="com.notifyvisitors.notifyvisitors.NotifyVisitorsApplication"
android:name="com.capacitor.notifyvisitors.NVApplication"
C. Set android:allowBackup to false.
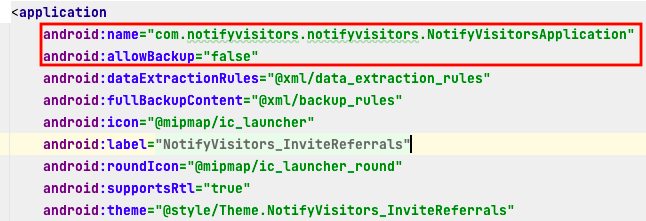
initialize application class and allow backup
Configure build.gradle
If have done FCM integration. These steps may exist in build.gradle files. So you can skip these steps.
Add the below code in build.gradle (module-level) at the bottom. If you don't have.
apply plugin: 'com.google.gms.google-services'
Add the below code in build.gradle (project-level). If you don't have.
buildscript {
dependencies {
// Add this line
classpath 'com.google.gms:google-services:4.0.1'
}
}
Updated 9 months ago