IOS Integration
Cordova Info
If your intregration is in Cordova. Then you have to skip this IOS Integration.
Now Add Notification Service Extension .
Goto the next page and Notification Service Extension on your project.
Cocoapods Install
Run this command from terminal
cd ios && pod install && cd ..
Configure info.plist
Source Code Edit
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
</dict>
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleURLName</key>
<string>$(PRODUCT_BUNDLE_IDENTIFIER)</string>
<key>CFBundleURLSchemes</key>
<array>
<string>YOUR_URL_SCHEME</string>
</array>
</dict>
</array>
<key>nvBrandID</key>
<integer>YOUR_BRANDID_COMES_HERE</integer>
<key>nvSecretKey</key>
<string>YOUR_SECRET_KEY_COMES_HERE</string>
<key>nvPushCategory</key>
<string>nvpush</string>
<key>nvViewAutoRedirection</key>
<true/> <!--OR--> <!-- <false/>-->
OR Property List Edit
.1 Add a new row by going to the menu and clicking Editor > Add Item. Setup a NSAppTransportSecurity
as a Dictionary
.
1.2 Added a Subkey called NSAllowsArbitraryLoads
as Boolean
and set its value to YES
as like the following image.
1.3 Add a new row again and set up a URL Types item by adding a new item. Expand the URL Types key, expand Item 0
, and add a new item, URL schemes. Fill in βappSchemeβ for Item 0 of URL schemes and your company identifier for the URL Identifier. Your file should resemble the image below when done.
1.4 Add a new row again and set up a nvBrandID
as Number
and fill this field with your BRANDID
1.5 Add a new row again and set up a nvSecretKey
as String and fill this field with your SECRET KEY
{8A991DED60F2186660EF8A337C30BDDE}
1.6 Add a new row again and set up a nvPushCategory
as String
and set itβs value nvpush
.
1.7 Add a new row again and set up a key nvViewAutoRedirection
as Boolean
set it YES
to enable auto redirection of your appβs ViewControllers
from sdk or set it NO
to handle redirections by your app.
1.9 Goto Signing & Capabilities
Tab and if βBackground Modes
β is not already added then click on the + symbol on the left corner of this tab and add Background Modes
make sure to select 2 checkboxes. If it is already added then make sure to select 2 checkboxes (i.e. Background fetch, Remote notifications).
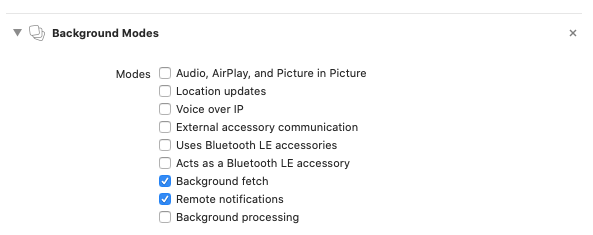
In the Signing & Capabilities
Tab and click on + symbol on the left corner of this tab and add Push Notifications
and if you are upgraded Xcode
and Push Notifications
was already added in previous version of Xcode
then remove Push Notifications
and add it again to configure push notification properly for the upgraded devices.
In the Signing & Capabilities
Tab if βApp Groups
β is not already added then click on + symbol on the left corner of this tab and add App Groups
and click on + sign and add a new app group give the group name as group.nv.{Your App Bundle Identifier
}.
Example : if Your Appβs Bundle Identifier is com.example.myapp
then the App Group must be named as group.nv.com.example.myapp
make sure this newly created app group must be checked (Turned on)
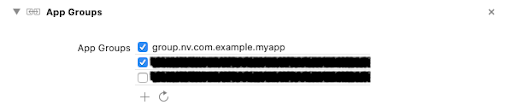
Swift (Bridging-Header)
If your project IOS platform is in Swift language. You have to create a Bridging-Header.h file. Add a new header file and name it with the following format. PROJECT_NAME-Bridging-Header.h
Import the below statement in PROJECT_NAME-Bridging-Header.h for accessing Native SDK Classes.
#import "notifyvisitors.h"
Make sure that the path of bridge-header.h file is included in build settings under βSwift compiler-code generationβ as:
Objective C bridging header: YOUR_PROJECT_NAME/YOUR_PROJECT_NAME-Bridging-Header.h
Import Header File
Import the below header file in your AppDelegate.m file in which SDK function is to be accessed.
#import "RNNotifyvisitors.h"
/* Objective C */
#import <flutter_notifyvisitors/NotifyvisitorsPlugin.h>
/* swift */
#import "NotifyvisitorsPlugin.h"
//you can import this statement on bridge-header file.
#import "notifyvisitors.h"
Initialise SDK
Initialize the SDK in the application didFinishLaunchingWithOptions
function.
[RNNotifyvisitors Initialize];
[RNNotifyvisitors RegisterPushWithDelegate: self App: application launchOptions: launchOptions];
/* Example */
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
RCTAppSetupPrepareApp(application);
RCTBridge *bridge = [self.reactDelegate createBridgeWithDelegate:self launchOptions:launchOptions];
// NotifyVisitors methods here
[RNNotifyvisitors Initialize];
[RNNotifyvisitors RegisterPushWithDelegate: self App: application launchOptions: launchOptions];
// ----------------------------
[super application:application didFinishLaunchingWithOptions:launchOptions];
return YES;
}
/* Objective C */
[NotifyvisitorsPlugin Initialize];
[NotifyvisitorsPlugin RegisterPushWithDelegate:self App:application launchOptions:launchOptions];
/* Example */
- (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[GeneratedPluginRegistrant registerWithRegistry:self];
// Override point for customization after application launch.
// NotifyVisitors methods here
[NotifyvisitorsPlugin Initialize];
[NotifyvisitorsPlugin RegisterPushWithDelegate:self App:application launchOptions:launchOptions];
// ----------------------------
return [super application:application didFinishLaunchingWithOptions:launchOptions];
}
/* = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = */
/* swift */
NotifyvisitorsPlugin.nvInitialize()
NotifyvisitorsPlugin.registerPush(withDelegate: self, app: application, launchOptions: launchOptions)
/* Example */
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
GeneratedPluginRegistrant.register(with: self)
// NotifyVisitors methods here
NotifyvisitorsPlugin.nvInitialize()
NotifyvisitorsPlugin.registerPush(withDelegate: self, app: application, launchOptions: launchOptions)
// ----------------------------
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
var nvMode:String? = nil
#if DEBUG
nvMode = "debug"
#else
nvMode = "live"
#endif
notifyvisitors.initialize(nvMode)
notifyvisitors.registerPush(withDelegate: self, app: application, launchOptions: launchOptions)
Add the following three functions inside your AppDelegate.m
file to handle the registering and receiving events of push notification
.
[RNNotifyvisitors application: application didRegisterForRemoteNotificationsWithDeviceToken: deviceToken];
[RNNotifyvisitors application: application didReceiveRemoteNotification: userInfo];
/* Example */
-(void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken{
[RNNotifyvisitors application: application didRegisterForRemoteNotificationsWithDeviceToken: deviceToken];
}
-(void)application:(UIApplication *)application didReceiveRemoteNotification: (NSDictionary *)userInfo {
[RNNotifyvisitors application: application didReceiveRemoteNotification: userInfo];
}
/* Objective C */
[NotifyvisitorsPlugin application:application didRegisterForRemoteNotificationsWithDeviceToken:deviceToken];
[NotifyvisitorsPlugin application:application didFailToRegisterForRemoteNotificationsWithError:error];
/* Example */
-(void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[NotifyvisitorsPlugin application:application didRegisterForRemoteNotificationsWithDeviceToken:deviceToken];
}
-(void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error{
[NotifyvisitorsPlugin application:application didFailToRegisterForRemoteNotificationsWithError:error];
}
/* = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = */
/* swift */
NotifyvisitorsPlugin.application(application, didFailToRegisterForRemoteNotificationsWithError: error)
NotifyvisitorsPlugin.application(application, didReceiveRemoteNotification: userInfo)
/* Example */
override func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) {
NotifyvisitorsPlugin.application(application, didFailToRegisterForRemoteNotificationsWithError: error)
}
override func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any]) {
NotifyvisitorsPlugin.application(application, didReceiveRemoteNotification: userInfo)
}
/* Swift */
notifyvisitors.didRegisteredNotification(application, deviceToken: deviceToken);
notifyvisitors.didReceiveRemoteNotification(userInfo: userInfo);
/* Example */
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
notifyvisitors.didRegisteredNotification(application, deviceToken: deviceToken);
}
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
notifyvisitors.didReceiveRemoteNotification(userInfo: userInfo);
}
Add the following method in applicationWillTerminate
In your AppDelegate.m
file
[RNNotifyvisitors applicationWillTerminate];
/* Example */
- (void)applicationWillTerminate:(UIApplication *)application{
[RNNotifyvisitors applicationWillTerminate];
}
/* Objective C */
[NotifyvisitorsPlugin applicationWillTerminate];
/* Example */
- (void) applicationWillTerminate:(UIApplication *)application{
[NotifyvisitorsPlugin applicationWillTerminate];
}
/* swift */
NotifyvisitorsPlugin.applicationWillTerminate()
/* Example */
override func applicationWillTerminate(_ application: UIApplication) {
NotifyvisitorsPlugin.applicationWillTerminate()
}
/* Swift */
notifyvisitors.applicationWillTerminate();
/* Example */
func applicationWillTerminate(_ application: UIApplication) {
notifyvisitors.applicationWillTerminate();
}
Rich Push Notification
Goto AppDelegate.h import UserNotifications framework and also add UNUserNotificationCenterDelegate
to support Rich Push Notifications.
#import <UserNotifications/UserNotifications.h>
@interface AppDelegate : UIResponder <UIApplicationDelegate, UNUserNotificationCenterDelegate>
@end
Now open AppDelegate.m
file and add the following delegate methods to handle rich push notifications.
# pragma mark UNNotificationCenter Delegate Methods
[RNNotifyvisitors willPresentNotification: notification withCompletionHandler: completionHandler];
[RNNotifyvisitors application:application didReceiveRemoteNotification:userInfo fetchCompletionHandler:completionHandler];
[RNNotifyvisitors didReceiveNotificationResponse: response];
/* Example */
- (void)userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification *)notification withCompletionHandler:(void (^)(UNNotificationPresentationOptions options))completionHandler {
[RNNotifyvisitors willPresentNotification: notification withCompletionHandler: completionHandler];
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler{
[RNNotifyvisitors application:application didReceiveRemoteNotification:userInfo fetchCompletionHandler:completionHandler];
}
-(void)userNotificationCenter:(UNUserNotificationCenter *)center didReceiveNotificationResponse:(UNNotificationResponse *)response withCompletionHandler:(void (^)(void))completionHandler {
[RNNotifyvisitors didReceiveNotificationResponse: response];
}
/* Objective C */
[NotifyvisitorsPlugin willPresentNotification:notification withCompletionHandler:completionHandler];
[NotifyvisitorsPlugin application:application didReceiveRemoteNotification:userInfo fetchCompletionHandler:completionHandler];
[NotifyvisitorsPlugin didReceiveNotificationResponse:response];
/* Example */
- (void)userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification *)notification withCompletionHandler:(void (^)(UNNotificationPresentationOptions options))completionHandler {
[NotifyvisitorsPlugin willPresentNotification:notification withCompletionHandler:completionHandler];
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center didReceiveNotificationResponse:(UNNotificationResponse *)response withCompletionHandler:(void (^)(void))completionHandler {
[NotifyvisitorsPlugin didReceiveNotificationResponse:response];
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler {
[NotifyvisitorsPlugin application:application didReceiveRemoteNotification:userInfo fetchCompletionHandler:completionHandler];
}
/* swift */
NotifyvisitorsPlugin.willPresent(notification, withCompletionHandler: completionHandler)
NotifyvisitorsPlugin.application(application, didReceiveRemoteNotification: userInfo)
NotifyvisitorsPlugin.didReceive(response)
/* Example */
override func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
NotifyvisitorsPlugin.willPresent(notification, withCompletionHandler: completionHandler)
}
override func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
NotifyvisitorsPlugin.application(application, didReceiveRemoteNotification: userInfo)
}
override func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
NotifyvisitorsPlugin.didReceive(response)
}
/* Swift */
notifyvisitors.willPresent( notification, withCompletionHandler: completionHandler)
notifyvisitors.didReceiveRemoteNotification(userInfo: userInfo);
notifyvisitors.pushNotificationActionData(from: response, autoRedirectOtherApps: true) { (pushData: NSMutableDictionary?) in
let userInfo: [AnyHashable : Any]?
if pushData!.count > 0 {
userInfo = (pushData as! [AnyHashable : Any])
} else {
userInfo = [:]
}
/* Example */
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
notifyvisitors.willPresent( notification, withCompletionHandler: completionHandler)
}
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
notifyvisitors.didReceiveRemoteNotification(userInfo: userInfo);
}
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse) async {
notifyvisitors.pushNotificationActionData(from: response, autoRedirectOtherApps: true) { (pushData: NSMutableDictionary?) in
let userInfo: [AnyHashable : Any]?
if pushData!.count > 0 {
userInfo = (pushData as! [AnyHashable : Any])
} else {
userInfo = [:]
}
NotificationCenter.default.post(name: Notification.Name.init("didReceiveResponse"), object: nil, userInfo: userInfo) ; }
}
Call the below method in your AppDelegate.m
that will check the deep linking
and open your app from URL Scheme
.
[RNNotifyvisitors openUrl:application openURL:url];
/* Example */
-(BOOL)application:(UIApplication*)application openURL:(NSURL*)url sourceApplication:(NSString*)sourceApplication annotation:(id)annotation {
[RNNotifyvisitors openUrl:application openURL:url];
return YES;
}
/* Objective C */
[NotifyvisitorsPlugin openUrl:application openURL:url];
/* Example */
-(BOOL)application:(UIApplication*)application openURL:(NSURL*)url sourceApplication:(NSString*)sourceApplication annotation:(id)annotation {
[NotifyvisitorsPlugin openUrl:application openURL:url];
return YES;
}
/* = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = */
/* swift */
NotifyvisitorsPlugin.openUrl(app, open: url)
/* Example */
override func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey : Any] = [:]) -> Bool {
NotifyvisitorsPlugin.openUrl(app, open: url)
return true
}
func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey: Any] = [:]) -> Bool {
notifyvisitors.openUrl(with: app, url: url);
return ApplicationDelegateProxy.shared.application(app, open: url, options: options)
}
Notification Service Extension
Add [Notification Service Extension] to support Rich media Attachments from iOS 10 push notifications. Kindly refer to our [Notification Service Extension] creation guide on the Next Page.
Updated 9 months ago