Push Notifications
Configure push notifications using NotifyVisitors.
1. Configure in NotifyVisitors Panel
a. Generate APNS Auth Key or APNS Certificate from Your Apple Developer Account:
You need to upload either “APNS Auth Key (recommended)” or “APNS Push Certificate” and some details from your Apple Developer Account into your notifyvisitos. For assistance to generate one of these certificates you can refer to the one of the following doc but we recommend you to use APNS Auth Key instead of APNS Push Certificate because it expires every year and you need to renew and reupload it each year.
b. Upload APNS Auth Key (.p8) or APNS Certificate (.pem) file in Notifyvisitors Account:
- After completing the previous step (a) you have either a .p8 file (recommended) or a .pem file (make sure to use the correct .pem file as described in the documentation because you will generate 3 different .pem files in that doc.). You need to upload this file in your NotifyVisitors account.
- Goto your NotifyVisitors panel and under any one of Analytics Product and now from the left side menu goto Settings >> App Push (under Channel section) >> iOS tab.
- Now make sure status should be Active if not make it active state.
- Select your “Authentication Type” as per the file created in the step (a). If you have created APNS Auth key (i.e. .p8 file) then select APNs Auth Key but if you have created APNS Push Certificate (i.e. .pem file) then select APNs Certificate option.
- Provide the others details as shown on this page from your Apple developer account and click on Save Changes button after providing the details. Make sure the given details must be correct and case sensitive so if details are mismatched then push may not be delivered to the device.
- Upload the .p8 file if you have selected “APNS Auth Key” or upload the correct .pem file (as described in doc) if you have selected the “APNS Certificate” option as created in previous steps.
- Now you can go to your app and configure push notifications as described in next step and after that step you can come back to your panel to create and send push notification from NotifyVisitors panel to your iOS devices.
2. Configure in Your App
After completing the Configure in Notifyvisitors Panel step, you need to configure push in your app which includes Register for push notifications, Enabling the Push Notifications Capability, Handling push and its click delegates, and Configure Notification Service Extension. Let’s discuss each step one by one in detail.
a. Register for push notifications:
It’s required to ask user permission first to enable push notifications on an iPhone device to do that goto your App’s AppDelegate.swift / AppDelegate.h file and import User Notifications and to handle push notifications click using delegate you need to use UNUserNotificationCenterDelegate in your AppDelegate file as shown below.
import UserNotifications
@main
class AppDelegate: UIResponder, UIApplicationDelegate, UNUserNotificationCenterDelegate
#import <UserNotifications/UserNotifications.h>
#import "notifyvisitors.h"
@interface AppDelegate : UIResponder <UIApplicationDelegate, UNUserNotificationCenterDelegate>
Add the following method in your AppDelegate.swift / AppDelegate.m file under didFinishLaunchingWithOptions method after SDK initialization as done in the Integration section.
notifyvisitors.registerPush(withDelegate: self, app: application, launchOptions: launchOptions)
Example:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
/* after notifyvisitors.initialize() method */
notifyvisitors.registerPush(withDelegate: self, app: application, launchOptions: launchOptions)
return true
}
[notifyvisitors RegisterPushWithDelegate: self App: application launchOptions: launchOptions];
Example:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
/* after notifyvisitors.initialize() method */
[notifyvisitors RegisterPushWithDelegate: self App: application launchOptions: launchOptions];
return YES;
}
Now add the following delegate method in your AppDelegate.swift / AppDelegate.m file to handle the registering events of push notification.
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
notifyvisitors.didRegisteredNotification(application, deviceToken: deviceToken)
}
func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) {
print("push registration failed due to the following error = \(error)" )
}
-(void)application:(UIApplication*)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[notifyvisitors DidRegisteredNotification:application deviceToken: deviceToken];
}
-(void)application:(UIApplication*)application didFailToRegisterForRemoteNotificationsWithError:(NSError*) error {
NSLog(@"push registration failed due to the following error = %@", error);
}
b. Configure Push in SignIn and Capabilities:
Goto Signing & Capabilities tab and click on + symbol on the left corner of this tab and add Push Notifications and if you are upgraded Xcode and Push Notifications was already added in previous version of Xcode then remove Push Notifications and add it again to configure push notification properly for the upgraded devices.

Again in the Signing & Capabilities tab if “Background Modes” is not already added then click on the + symbol again and add Background Modes make sure to select 2 checkboxes. If it is already added then make sure to select 2 checkboxes (i.e. Background fetch, Remote notifications) as shown in the screenshot below.
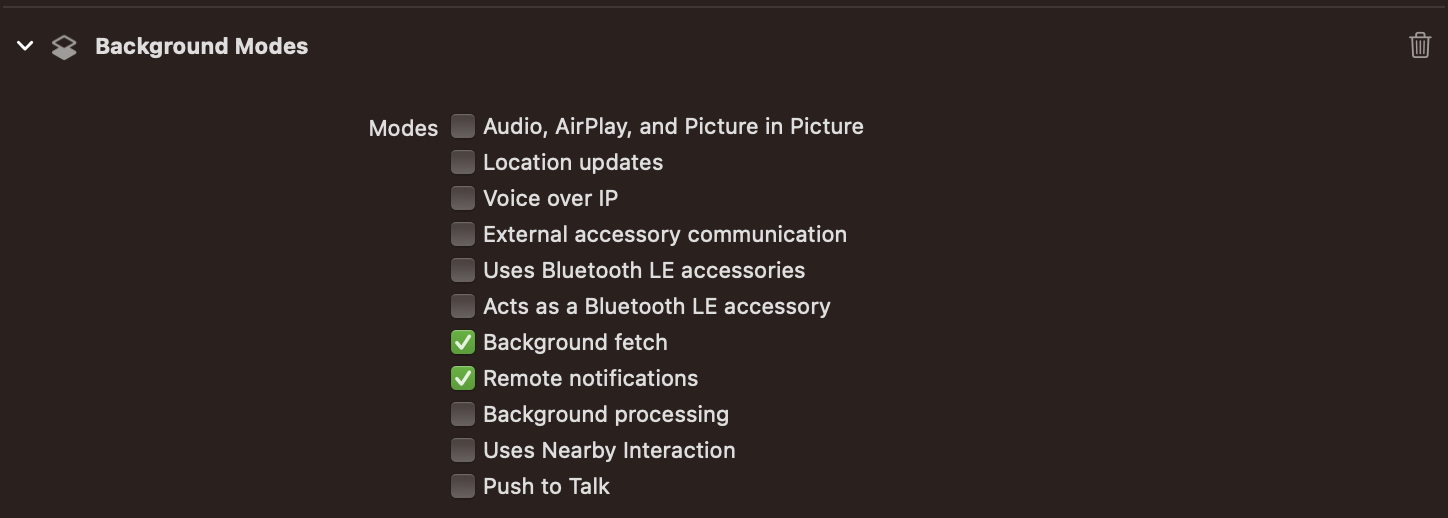
c. Handling Push Notifications Delegates & It’s Clicks:
Now by using UNUserNotificationCenterDelegate protocol in your AppDelegate.swift / AppDelegate.m file you need to implement below given delegate methods to handle push receiving (while app is in foreground) and push click events as described below.
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
notifyvisitors.willPresent(notification, withCompletionHandler: completionHandler)
}
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
notifyvisitors.didReceiveRemoteNotification(userInfo, fetchCompletionHandler: completionHandler)
}
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
notifyvisitors.pushNotificationActionData(from: response, autoRedirectOtherApps: true) { (pushData : NSMutableDictionary?) in
print("pushData for further action = \(pushData!)")
/* Here the data received in the pushData variable is required to execute further action and redirect to the desired ViewController. When the target is set to NavigateInApp or Universal Link, those conditions must be handled from here by using target values 0 and 6, respectively.
If the second parameter for "autoRedirectOtherApps" is false, all push click actions must be handled from here by using pushData values,
whereas if true, only NavigateInApp (target = 0) and Universal link (target = 6) values should be handled from here. */
completionHandler()
}
}
-(void)userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification *)notification withCompletionHandler:(void (^)(UNNotificationPresentationOptions))completionHandler {
[notifyvisitors willPresentNotification: notification withCompletionHandler:completionHandler];
}
-(void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler {
[notifyvisitors didReceiveRemoteNotification: userInfo fetchCompletionHandler: completionHandler];
}
-(void)userNotificationCenter:(UNUserNotificationCenter*)center didReceiveNotificationResponse: (UNNotificationResponse*)response withCompletionHandler:(void (^)(void))completionHandler {
[notifyvisitors PushNotificationActionDataFromResponse: response AutoRedirectOtherApps: YES clickResponseData:^(NSMutableDictionary *pushData) {
NSLog(@"pushData for further action = %@", pushData);
/* Here the data received in the pushData variable is required to execute further action and redirect to the desired ViewController. When the target is set to NavigateInApp or Universal Link, those conditions must be handled from here by using target values 0 and 6, respectively.
If the second parameter for "autoRedirectOtherApps" is false, all push click actions must be handled from here by using pushData values,
whereas if true, only NavigateInApp (target = 0) and Universal link (target = 6) values should be handled from here. */
completionHandler();
}];
}
d. Enable Rich Media Content by using Notification Service Extension:
Till the above steps are completed you will be able to receive only text content (i.e. title and message text) in push notifications and also delivery count can not be tracked in NotifyVistors panel. You need to configure Notification Service Extension along with AppGroup properly to complete Push integration and it will enable your push notification to receive rich media (image, audio or video) and also NotifyVisitors used it to enable you to add Action buttons, badge counts and to track delivery counts in your NotifyVisitors panel.You need to refer to our Notification Service Extension guide for detailed configuration steps for the same.
Updated about 1 month ago