In-app Notifications
Pass tokens and customer rules to show in-app notifcations.
Configure target rules and pass tokens to personalized experience and custom rules to show in-app notifications.
In-app messaging includes both Banners and Surveys. They are displayed on the basis of user ViewControllers or custom rules.
Every event occurs in the context of a ViewController. Every view controller can be associated with some contextual data, which can be used as targeting rules for In-app Messaging.
To display banners or surveys in your app, integrate the following code, which should be used only once per ViewController.
notifyvisitors.show(UserTokensDictionary, customRule: customRuleDictionary)
Example:
notifyvisitors.show(nil, customRule: nil)
[notifyvisitors Show: UserTokensDictionary CustomRule: customRuleDictionary];
Example:
[notifyvisitors Show: nil CustomRule: nil];
If user token or custom rule values are not available then it can be passed as nil value as shown in above example.
Dynamic Tokens / User Token (NSMutableDictionary)
Dynamic tokens are used to show personalized content in Notification messages in real time.
let tokens = [:]
tokens["customer_name"] = "John"
tokens["deal_name"] = "Deal of the day"
tokens["discount_percent"] = "20"
let finalUserTokens = NSMutableDictionary(dictionary: tokens)
notifyvisitors.show(finalUserTokens, customRule: nil)
NSMutableDictionary *tokens = [[NSMutableDictionary alloc] init];
[tokens setObject: @"John" forKey: @"customer_name"];
[tokens setObject: @"Deal of the day" forKey: @"deal_name"];
[tokens setObject: @"20" forKey: @"discount_percent"];
[notifyvisitors Show: finalUserTokens CustomRule: nil];
Custom Rule (NSMutableDictionary)
This data can be used in configuring targeting rules for the inApp notifications.
let customRule = [:]
customRule["page_id"] = "dashboard"
customRule["category"] = "fashion"
customRule["price"] = "2000"
let finalCustomRule = NSMutableDictionary(dictionary: customRule)
notifyvisitors.show(finalUserTokens, customRule: finalCustomRule)
NSMutableDictionary *customRule = [[NSMutableDictionary alloc] init];
[customRule setObject: @"dashboard" forKey: @"page_id"];
[customRule setObject: @"fashion" forKey: @"category"];
[customRule setObject: @"2000" forKey: @"price"];
[notifyvisitors Show: finalUserTokens CustomRule: finalCustomRule];
In-app Notification on UIScrollView Scroll Percentage
If you are using UIScrollView and wants to show a banner/survey after a given scroll percentage then in notifyvisitors panel set the percentage value under targeting rule of you in-app notification setting and call the show() method inside your viewdidLoad() method and call the below given method inside your UIScrollView’s delegate “scrollViewDidScroll” method.
func scrollViewDidScroll(_ scrollView: UIScrollView) {
notifyvisitors.scrollViewDidScroll(scrollView)
}
-(void)scrollViewDidScroll:(UIScrollView *)scrollView {
[notifyvisitors scrollViewDidScroll: scrollView];
}
Note
Once you provide a scroll percentage value in your in-app notifications setting in your panel and call show() method inside your viewdidLoad() method then that notification will wait to show till user scroll to the given percentage and completion of scrolled percentage will be tracked using the scrollViewDidScroll() delegate method as described in above example.
In-app Notifications Response Callback
If you want to get callback data whenever a user clicks on an in-app banner or fills out a survey, you can use the SDK's event callback. It will receive data in the same callback method if you use event tracking and its callback on the same page. However, if you don't use the event callback on the same page, then you can get the event callback in the app using notifyvisitorsDelegate. As shown below, add notifyvisitorsDelegate to the viewController you are triggering the show() method in.
class ViewController: notifyvisitorsDelegate
#import "notifyvisitors.h"
@interface ViewController : UIViewController <notifyvisitorsDelegate>
Now set the delegate in the viewdidLoad() method of your viewController file before triggering the show() method.
override func viewDidLoad() {
super.viewDidLoad()
notifyvisitors.sharedInstance()?.delegate = self
}
- (void)viewDidLoad {
[super viewDidLoad];
[notifyvisitors sharedInstance].delegate = self;
}
Add the following delegate method into the viewController file to receive the callbacks of events performed.
func notifyvisitorsGetEventResponse(userInfo: [AnyHashable : Any]? = nil) {
print("event callback response = \(userInfo ?? [:])")
}
-(void)NotifyvisitorsGetEventResponseWithUserInfo:(NSDictionary *)userInfo {
NSLog(@"event callback response = %@", userInfo);
}
This callback response will provide you output in NSDictionary format. A sample response given below for your reference.
In-app notification event sample response:
{
"status" : "success",
"message" : "Survey submitted successfully",
"callbackType" : "survey",
"eventName" : "Survey Submit",
"attributes" : {
"notificationID" : "2628",
"rating" : "9"
},
"type" : 14.1
}
In the above output, the parameters have different values depending on the scenario that occurs when displaying banners or surveys. Below are the different values you can get in the callback:
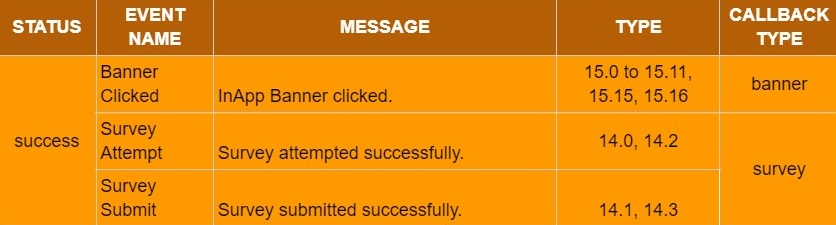
Navigate to webpage through in-app banner
The undermentioned function can be triggered to navigate to the webpage:
window.webkit.messageHandlers.nvOpenWeb.postMessage("Link/User Token")
Updated about 1 year ago