Notification Runtime Permission
Android 13 (API level 33) and higher supports runtime permission for sending non-exempt (including Foreground Services (FGS)) notifications from an app. We recommend targeting Android 13 or higher as soon as possible to benefit from this feature's additional control and flexibility. Click here to learn more about push prompt notification permission.
Note
This feature will be available from the SDK Version: 5.3.10
How to configure the prompt in your app
SDK provides you three options to deal with the prompt configuration.
Option 1: Your own designed popup with your logic
Whenever the user consents to receive push notifications via a system-generated permission prompt, then call the undermtioned function by means of following the step by step procedure delineated below. This particular function will inform the NotifyVisitors SDK that the user has granted the permission to receive the notifications.
Step 1: Import Statement
import com.notifyvisitors.notifyvisitors.NotifyVisitorsApi;
import com.notifyvisitors.notifyvisitors.NotifyVisitorsApi
Step 2: Add the undermentioned code whenever you get a response from the user
NotifyVisitorsApi.getInstance(activityContext).enablePushPermission(isAllowed (boolean));
NotifyVisitorsApi.getInstance(activityContext).enablePushPermission(isAllowed (boolean))
Remember
Do not call this function in case the user denies receiving push notifications.
isAllowed: A boolean type parameter that tells NotifyVisitors Android SDK whether the user accepted or denied the permission. Pass TRUE when the user allows it and pass FALSE when it is denied.
Option 2: SDK’s designed pop-up with logic
An illustration of the default UI of the SDK’s Push Permission Prompt has been provided below. Customizations are available as shown in the screenshots. We have two template designs for permission prompts. Each contains four parts viz. title, description, a positive option as button 1, and a negative option as button 2.
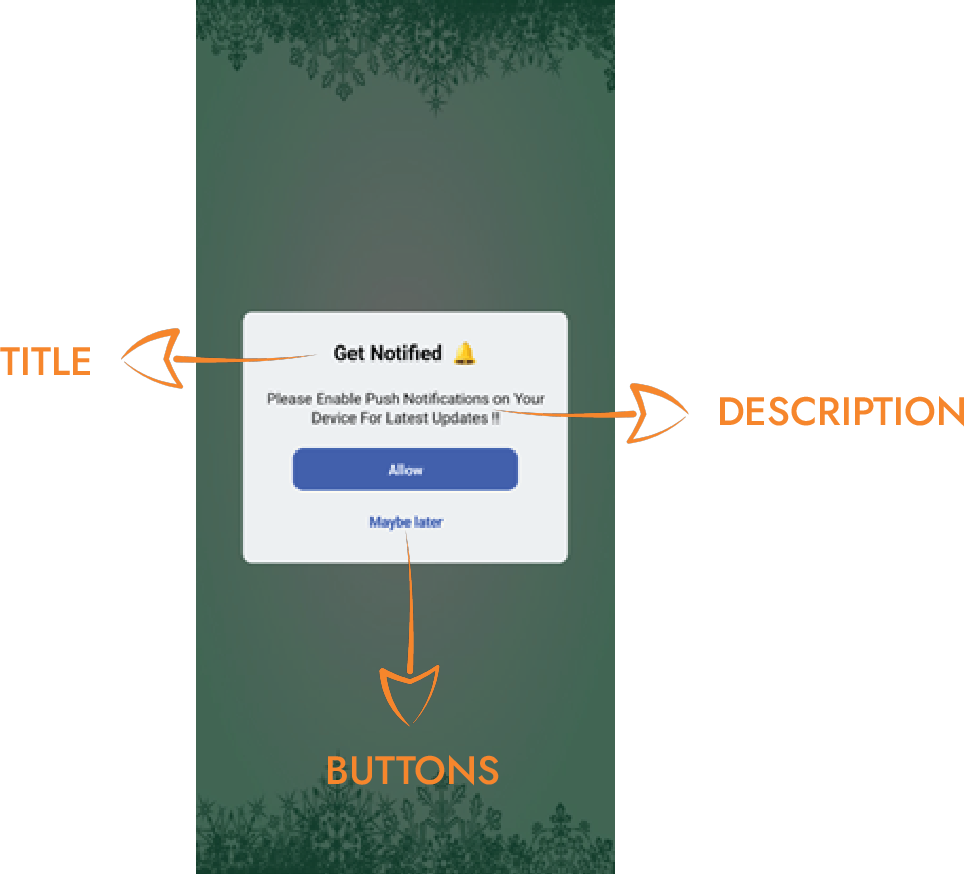
[ Template 1 ]
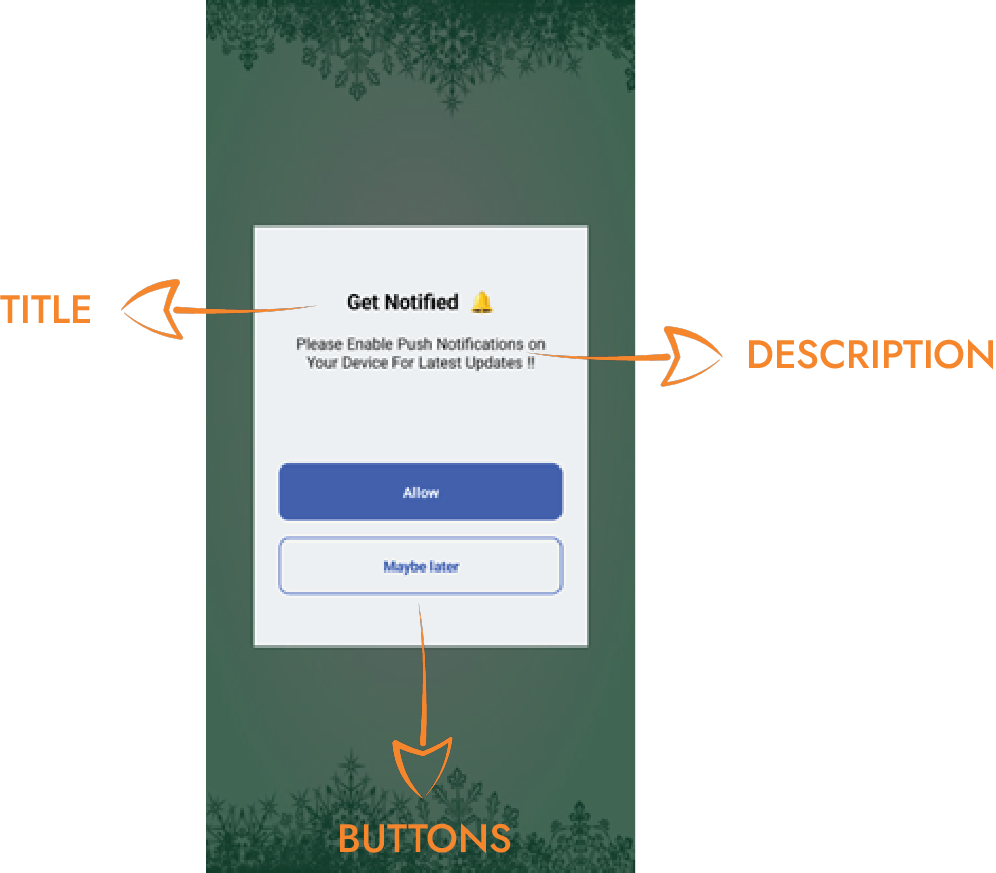
[ Template 0 ]
To achieve this follow the undermentioned steps:
Step 1 - Add the undermentioned import statements
import com.notifyvisitors.notifyvisitors.NotifyVisitorsApi;
import com.notifyvisitors.notifyvisitors.interfaces.OnPushRuntimePermission;
import com.notifyvisitors.notifyvisitors.permission.NVPopupDesign;
import com.notifyvisitors.notifyvisitors.NotifyVisitorsApi
import com.notifyvisitors.notifyvisitors.interfaces.OnPushRuntimePermission
import com.notifyvisitors.notifyvisitors.permission.NVPopupDesign
Step 2 - Add the undermentioned code to your activity
NVPopupDesign design = new NVPopupDesign();
design.setTitle("text");
design.setTitleTextColor("color_in_hexcode_format");
design.setDescription("text");
design.setDescriptionTextColor("color_in_hexcode_format");
design.setBackgroundColor("color_in_hexcode_format");
design.setNumberOfSessions(count_as_integer);
design.setResumeInDays(days_as_integer);
design.setNumberOfTimesPerSession(count_as_integer);
design.setTemplateID(integer);
design.setButtonOneBorderColor("color_in_hexcode_format");
design.setButtonOneBorderRadius(radius_as_integer);
design.setButtonOneText("text");
design.setButtonOneTextColor("color_in_hexcode_format");
design.setButtonOneBackgroundColor("color_in_hexcode_format");
design.setButtonTwoText("text");
design.setButtonTwoTextColor("color_in_hexcode_format");
design.setButtonTwoBackgroundColor("color_in_hexcode_format");
design.setButtonTwoBorderColor("color_in_hexcode_format");
design.setButtonTwoBorderRadius(radius_as_integer);
NotifyVisitorsApi.getInstance(activityContext).activatePushPermissionPopup(design, new OnPushRuntimePermission() {
@Override
public void getPopupInfo(JSONObject result) {
//do your task here
}
});
val design = NVPopupDesign()
design.setTitle("text")
design.setTitleTextColor("color_in_hexcode_format")
design.setDescription("text")
design.setDescriptionTextColor("color_in_hexcode_format")
design.setBackgroundColor("color_in_hexcode_format")
design.setNumberOfSessions(count_as_integer)
design.setResumeInDays(days_as_integer);
design.setNumberOfTimesPerSession(count_as_integer)
design.setTemplateID(integer)
design.setButtonOneBorderColor("color_in_hexcode_format")
design.setButtonOneBorderRadius(radius_as_integer)
design.setButtonOneText("text")
design.setButtonOneTextColor("color_in_hexcode_format")
design.setButtonOneBackgroundColor("color_in_hexcode_format")
design.setButtonTwoText("text")
design.setButtonTwoTextColor("color_in_hexcode_format")
design.setButtonTwoBackgroundColor("color_in_hexcode_format")
design.setButtonTwoBorderColor("color_in_hexcode_format")
design.setButtonTwoBorderRadius(radius_as_integer)
NotifyVisitorsApi.getInstance(this)
.activatePushPermissionPopup(design, object : OnPushRuntimePermission {
override fun getPopupInfo(it: JSONObject) {
//do your task here
}
})
Note
The function above has two parameters namely: numberOfSessions and resumeInDays. These two parameters will handle the flow of SDK’s push prompt permission (not the system’s permission dialog).
numberOfSessions → This parameter takes integer as input. In NV SDK, new or next session is created either on first app launch or after 30 minutes of user’s inactivity. So, if you set 3, then prompt will be displayed for next 3 sessions including current session in case user denies the prompt in every attempt.
resumeInDays → This parameter takes integer as input. When numberOfSessions set by you is completed then resumeInDays parameter will work. This is used to control the time period in days after which you intend to show the prompt again to the user. So, if you set 10 then prompt will remain hidden for 10 days and from the 11th day onwards it will get displayed according to the condition set in numberOfSessions parameter.
Title
Title of Permission Prompt. It is multi-line. Its default value is 'Get Notified \uD83D\uDD14'.
design.setTitle("Get Notified \uD83D\uDD14");
design.setTitle("Get Notified \uD83D\uDD14")
Additional Customizations
To change the title’s text style to such as italic, normal, etc. you can do so from here. The default value is bold. Four values can be passed here: bold, italic, normal, and bold-italic.
<string name="nv_prppTitleTextStyle">bold</string>
To change the title’s text size you can change it from here. The default value is 22.
<integer name="nv_prppTitleTextSize">22</integer>
TitleTextColor
Title’s text color of Permission Prompt. Its default value is '#000000'.
design.setTitleTextColor("#000000");
design.setTitleTextColor("#000000")
Description
Description of Permission Prompt. It is multi-line. Its default value is 'Please Enable Push Notifications on Your Device For Latest Updates !!'.
design.setDescription("Enable notifications on your device for push notifications");
design.setDescription("Enable notifications on your device for push notifications")
Additional Customizations
To change the description’s text style such as italic, normal, etc. you can change it from here. The default value is bold. Four values can be passed here: bold, italic, normal, and bold-italic.
<string name="nv_prppDescTextStyle">bold</string>
To change the description’s text size you can change it from here. The default value is 18.
<integer name="nv_prppDescTextSize">18</integer>
[ Only for Template 1 ] To handle the description’s top and bottom margin you can do it from here.
<integer name="nv_prppDescTopMargin">20</integer>
<integer name="nv_prppDescBottomMargin">20</integer>
DescriptionTextColor
Description’s text color of Permission Prompt. Its default value is '#000000'.
design.setDescriptionTextColor("#000000");
design.setDescriptionTextColor("#000000")
BackgroundColor
Permission Prompt’s background color. Its default value is '#EBEDEF'. The value should be in hex color code format.
design.setBackgroundColor("#EBEDEF");
design.setBackgroundColor("#EBEDEF")
Additional Customizations [ Only for Template 1 ]
To handle the main layout’s or background’s padding, do it from here.
<integer name="nv_prppMainTopPadding">25</integer>
<integer name="nv_prppMainBottomPadding">20</integer>
<integer name="nv_prppMainLeftPadding">10</integer>
<integer name="nv_prppMainRightPadding">10</integer>
NumberOfSessions
This parameter takes integer as input. In NV SDK, new or next session is created either on first app launch or after 30 minutes of user's inactivity. So, if you set 3, then the prompt will be displayed for next 3 sessions including the current session, in case user denies the prompt in every attempt.
Default value is 1.
design.setNumberOfSessions(2);
design.setNumberOfSessions(2)
ResumeInDays
This parameter takes integer as input. When NumberOfSessions set by you is completed then ResumeInDays parameter will work. This is used to control the time period in days after which you intend to show the prompt again to the user. So, if you set 10 then prompt will remain hidden for 10 days and from the 11th day onwards it will get displayed according to the condition set in the NumberOfSessions parameter.
Default value is 1.
design.setResumeInDays(1);
design.setResumeInDays(1)
NumberOfTimesPerSession
This parameter takes an integer as input. Its main purpose is for testing. We recommend not to use this parameter until it's required. It will help you show the prompt 'n' times in a single session. For example, if you set the value as 5 then the prompt will be displayed 5 times in a session and 1 time in each app launch.
Default value is 1.
design.setNumberOfTimesPerSession(5);
design.setNumberOfTimesPerSession(5)
ButtonOneText
Text of Button One of Permission Prompt. It is also called a Positive Button. Whenever this button is pressed it will show the system’s permission prompt. The default value is 'Allow'.
design.setButtonOneText("Allow");
design.setButtonOneText("Allow")
Additional Customizations
To change the button one’s text style to italic, normal, etc., you can change it from here. The default value is bold. Four values can be passed here: bold, italic, normal, and bold-italic.
<string name="nv_prppBtnOneTextStyle">bold</string>
ButtonOneBorderColor
Border Color of 'Button One' of Permission Prompt. The default value is '#6db76c'.
design.setButtonOneBorderColor("#6db76c");
design.setButtonOneBorderColor("#6db76c")
ButtonOneBorderColor
Border Radius (creates curved edges) of 'Button One' of Permission Prompt. The default value is 25.
design.setButtonOneBorderRadius(25);
design.setButtonOneBorderRadius(25)
Additional Customizations
Border Width of 'Button One' of Permission Prompt. The default value is 1.
<integer name="nv_prppBtnOneBorderWidth">1</integer>
[ Ony for Template 1 ] Height and Width of 'Button One' of Permission Prompt.
<integer name="nv_prppBtnOneWidth">220</integer>
<integer name="nv_prppBtnOneHeight">40</integer>
ButtonOneTextColor
Text color of 'Button One' of Permission Prompt. The default value is '#FFFFFF'.
design.setButtonOneTextColor("#FFFFFF");
design.setButtonOneTextColor("#FFFFFF")
ButtonOneBackgroundColor
Background color of 'Button One' of Permission Prompt. The default value is '#2254F5'.
design.setButtonOneBackgroundColor("#2254F5");
design.setButtonOneBackgroundColor("#2254F5")
ButtonTwoText
Text of 'Button Two' of Permission Prompt. It is also called a 'Negative Button'. Whenever this button is pressed it will cancel the permission prompt. The default value is 'Maybe later'.
design.setButtonTwoText("Maybe later");
design.setButtonTwoText("Maybe later")
Additional Customizations
To change the button two’s text style to italic, normal, etc., you can change it from here. The default value is bold. Four values can be passed here: bold, italic, normal, and bold-italic.
<string name="nv_prppBtnTwoTextStyle">bold</string>
ButtonTwoTextColor
Text Color of 'Button Two' of Permission Prompt. The default value is '#2254F5'.
design.setButtonTwoTextColor("#2254F5");
design.setButtonTwoTextColor("#2254F5")
ButtonTwoBackgroundColor
Background Color of 'Button Two' of Permission Prompt. The default value is '#EBEDEF'.
design.setButtonTwoBackgroundColor(“#EBEDEF”);
design.setButtonTwoBackgroundColor(“#EBEDEF”)
ButtonTwoBorderColor
Border Color of 'Button Two' of Permission Prompt. The default value is '#2254F5'.
design.setButtonTwoBorderColor(“#2254F5”);
design.setButtonTwoBorderColor(“#2254F5”)
ButtonTwoBorderRadius
Border Radius (creates curved edges) of 'Button Two' of Permission Prompt.
design.setButtonTwoBorderRadius(25);
design.setButtonTwoBorderRadius(25)
Additional Customizations
[ Only for Template 0 ] Border Width of 'Button Two' of Permission Prompt. The default value is 3.
<integer name="nv_prppBtnTwoBorderWidthTemp0">3</integer>
[ Only for Template 1 ] Border Width of 'Button Two' of Permission Prompt. The default value is 0.
<integer name="nv_prppBtnTwoBorderWidthTemp1">0</integer>
[ Only for Template 1 ] Height and Width of 'Button Two' of Permission Prompt.
<integer name="nv_prppBtnTwoWidth">220</integer>
<integer name="nv_prppBtnTwoHeight">40</integer>
[ Only for Template 1 ] Top Margin of 'Button Two' of Permission Prompt.
<integer name="nv_prppBtnTwoTopMargin">10</integer>
TemplateID
Template ID of Permission Prompt. The default template is 1.
design.setTemplateID(1);
design.setTemplateID(1)
Step 3 - Example
Below is the example with 'Template 1' customizations.
NVPopupDesign design = new NVPopupDesign();
design.setTitle("\"NV\" Would you like to Send You Notifications");
design.setTitleTextColor("#000000");
design.setDescription("Notifications may include alerts, sounds, and icon badges. These can be configured in Settings.");
design.setDescriptionTextColor("#000000");
design.setBackgroundColor("#DBFEEB");
design.setNumberOfSessions(3);
design.setResumeInDays(1);
design.setNumberOfTimesPerSession(40);
design.setButtonOneBorderRadius(25);
design.setTemplateID(1);
design.setButtonTwoBackgroundColor("#DBFEEB");
design.setButtonOneBorderColor("#3A9B72");
design.setButtonOneText("Allow");
design.setButtonOneTextColor("#ffffff");
design.setButtonOneBackgroundColor("#3A9B72");
design.setButtonTwoText("Deny");
design.setButtonTwoTextColor("#3A9B72");
design.setButtonTwoBackgroundColor("#DBFEEB");
design.setButtonTwoBorderColor("#3A9B72");
design.setButtonTwoBorderRadius(25);
NotifyVisitorsApi.getInstance(activityContext).activatePushPermissionPopup(design, new OnPushRuntimePermission() {
@Override
public void getPopupInfo(JSONObject result) {
try {
Log.d("App", "Prompt Response => " + result);
} catch (Exception e) {
e.printStackTrace();
}
}
});
val design = NVPopupDesign()
design.setTitle("\"NV\" Would you like to Send You Notifications")
design.setTitleTextColor("#000000")
design.setDescription("Notifications may include alerts, sounds, and icon badges. These can be configured in Settings.")
design.setDescriptionTextColor("#000000")
design.setBackgroundColor("#DBFEEB")
design.setNumberOfSessions(3)
design.setResumeInDays(1)
design.setNumberOfTimesPerSession(40)
design.setButtonOneBorderRadius(25)
design.setTemplateID(1)
design.setButtonTwoBackgroundColor("#DBFEEB")
design.setButtonOneBorderColor("#3A9B72")
design.setButtonOneText("Allow")
design.setButtonOneTextColor("#ffffff")
design.setButtonOneBackgroundColor("#3A9B72")
design.setButtonTwoText("Deny")
design.setButtonTwoTextColor("#3A9B72")
design.setButtonTwoBackgroundColor("#DBFEEB")
design.setButtonTwoBorderColor("#3A9B72")
design.setButtonTwoBorderRadius(25)
NotifyVisitorsApi.getInstance(activityContext).activatePushPermissionPopup(
design) { result: JSONObject? ->
try {
if (result != null) {
Log.d("App", "Prompt Response => $result")
}
} catch (e: Exception) {
e.printStackTrace()
}
}
Output with Callback
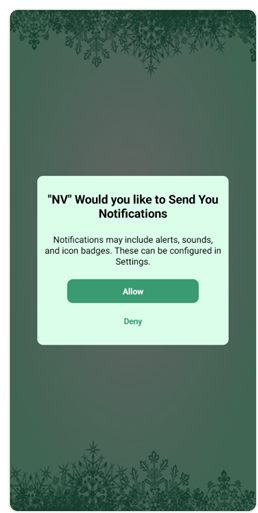
{
"status":"success",
"message":"Popup launched. User granted permission."
}
Option 3: Activate Native Permission Prompt
If you want to opt for a native permission prompt or system-designed prompt from NotifyVisitors SDK, you can add the below code to your activity.
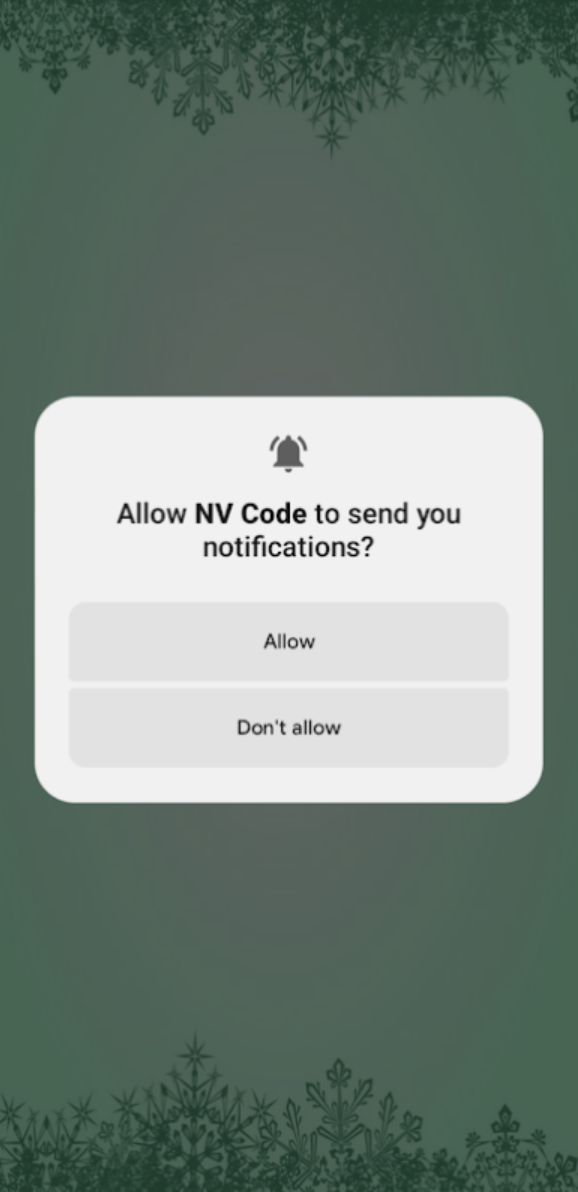
Keep in mind that you can request permission from the user a maximum of two times. If the user declines both times, you won't have the opportunity to ask again. The only option available to you is to guide the user to the notification permission settings page of your app.
NotifyVisitorsApi.getInstance(activityContext).nativePushPermissionPrompt(new OnPushRuntimePermission() {
@Override
public void getPopupInfo(JSONObject result) {
//do your work here
}
});
NotifyVisitorsApi.getInstance(activityContext)
.nativePushPermissionPrompt(object : OnPushRuntimePermission {
override fun getPopupInfo (result: JSONObject) {
//do your work here
}
})
Here are some of the 'callbacks' you can receive in the function mentioned above in Option 2 and Option 3.
STATUS
|
MESSAGE
|
Success
|
Push permission is already active on this device.
|
Pop-up launched. User granted permission.
|
|
Push Notification Settings is enabled by default from OS below Android 12.
|
|
Push Notification Settings is ON.
|
|
Fail
|
Pop-up launched. User denied SDK's custom popup permission.
|
Pop-up launched. User denied permission.
|
|
Error
|
Something went wrong with error --- <error>
|
Updated 4 months ago