Notification Runtime Permission (Android)
Android 13 (API level 33) and higher supports runtime permission for sending non-exempt (including Foreground Services (FGS)) notifications from an app.
We recommend targeting Android 13 or higher as soon as possible to benefit from this feature's additional control and flexibility. Click here to learn more about push prompt notification permission.
How to configure the prompt in your app
The SDK offers you three different ways to manage the prompt configuration.
Import Package
import { PushPromptInfo } from 'react-native-notifyvisitors';
Option 1: Your own designed popup with your logic
Whenever the user consents to receive push notifications via a system-generated permission prompt, then call the undermtioned function by means of following the step by step procedure delineated below. This particular function will inform the NotifyVisitors SDK that the user has granted the permission to receive the notifications.
Notifyvisitors.checkPushActive(true);
isAllowed: A boolean type parameter that tells NotifyVisitors Android SDK whether the user accepted or denied the permission. Pass TRUE when the user allows it and pass FALSE when it is denied.
Option 2: SDK’s designed pop-up with logic
An illustration of the default UI of the SDK’s Push Permission Prompt has been provided below. Customizations are available as shown in the screenshots. We have two template designs for permission prompts. Each contains four parts viz. title, description, a positive option as button 1, and a negative option as button 2.
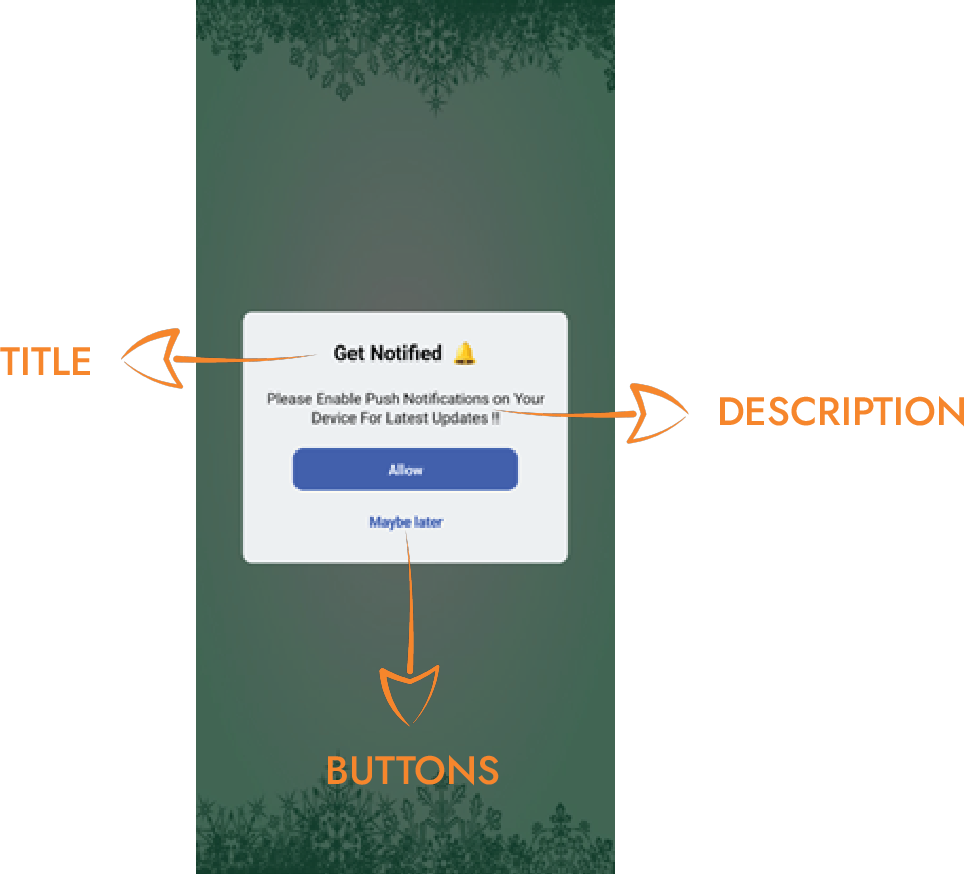
[ Template 1 ]
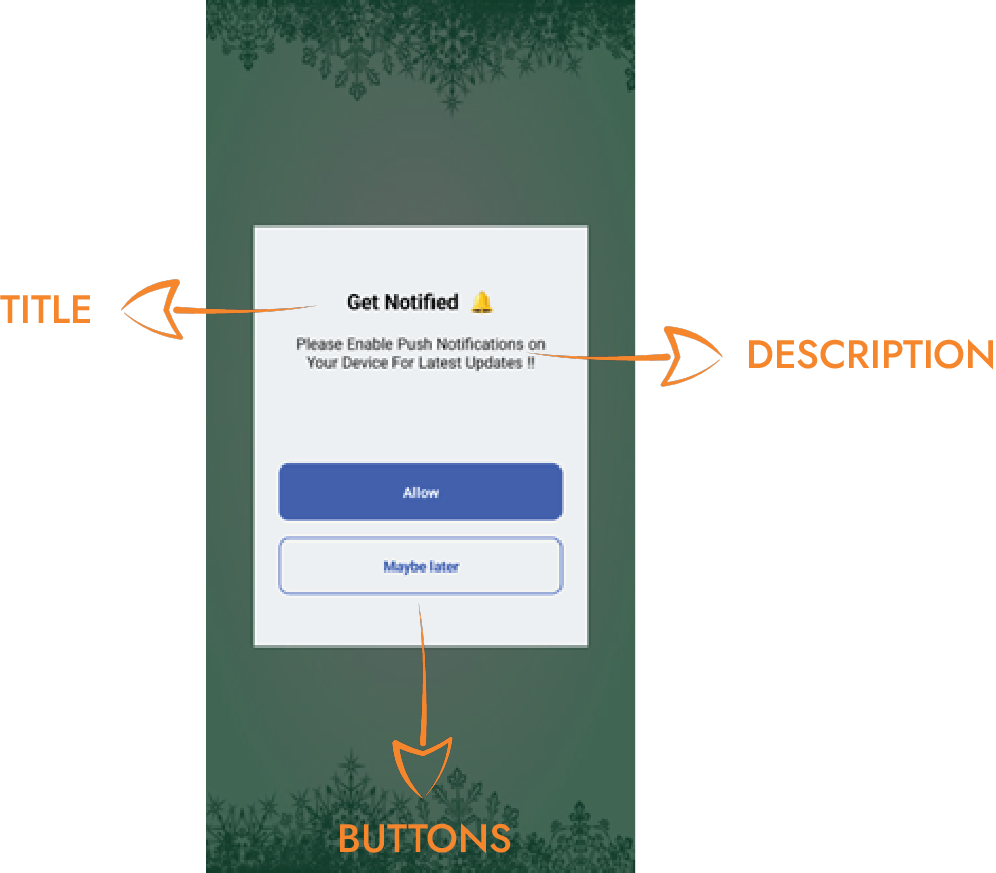
[ Template 0 ]
To achieve this follow the undermentioned steps:
Add the undermentioned code to your class
let design = new PushPromptInfo();
design.title = "Test Title";
design.titleTextColor = "#000000";
design.description = "Enable Push Notifications on Your Device !!";
design.descriptionTextColor = "#000000";
design.backgroundColor = "#EBEDEF";
design.buttonOneBorderColor = "#6db76c";
design.buttonOneBackgroundColor = "#26a524";
design.buttonOneBorderRadius = "0";
design.buttonOneText = "Allow";
design.buttonOneTextColor = "#FFFFFF";
design.buttonTwoText = "Cancel";
design.buttonTwoTextColor = "#FFFFFF";
design.buttonTwoBackgroundColor = "#FF0000";
design.buttonTwoBorderColor = "#6db76c";
design.buttonTwoBorderRadius = "0";
design.numberOfSessions = "3";
design.resumeInDays = "1";
design.numberOfTimesPerSession = "6";
Notifyvisitors.pushPermissionPrompt(design, function (response: any) {
//do your work here
});
Notice that there are two parameters in the function above namely numberOfSessions and resumeInDays. These two parameters will handle the flow of SDK’s push prompt permission (not system’s permission dialog).
numberOfSessions → This parameter takes integer as input. In NV SDK, new or next session is created either on first app launch or after 30 minutes of user’s inactivity. So, if you set 3, then prompt will be displayed for next 3 sessions including current session in case user denies the prompt in every attempt.
resumeInDays → This parameter takes integer as input. When numberOfSessions set by you is completed then resumeInDays parameter will work. This is used to control the time period in days after which you intend to show the prompt again to the user. So, if you set 10 then prompt will remain hidden for 10 days and from the 11th day onwards it will get displayed according to the condition set in numberOfSessions parameter.
Option 3: Activate Native Permission Prompt
If you want to opt for a native permission prompt or system-designed prompt from NotifyVisitors SDK, you can add the below code to your activity.
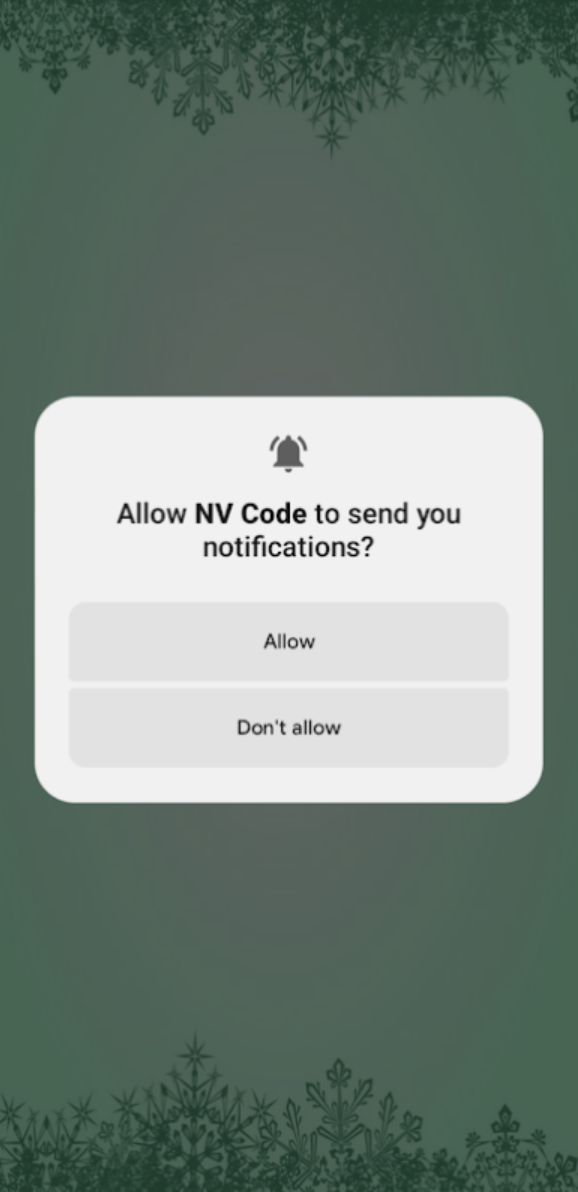
Keep in mind that you can request permission from the user a maximum of two times. If the user declines both times, you won't have the opportunity to ask again. The only option available to you is to guide the user to the notification permission settings page of your app.
Notifyvisitors.nativePushPermissionPrompt(function(callback: JSON){
//do your work here
});
Output with callback
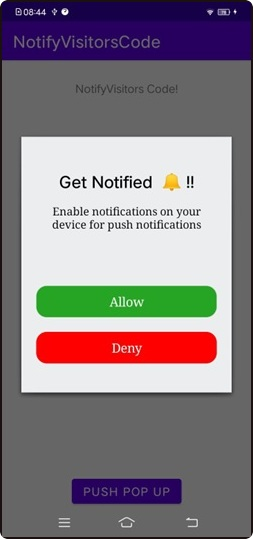
{
"status":"success",
"message":"Popup launched. User granted permission."
}
Callback responses
Here are some of the 'callbacks' you can receive in the function mentioned above in Option 2 and Option 3.
STATUS
|
MESSAGE
|
Success
|
Push permission is already active on this device.
|
Pop-up launched. User granted permission.
|
|
Push Notification Settings is enabled by default from OS below Android 12.
|
|
Push Notification Settings is ON.
|
|
Fail
|
Pop-up launched. User denied SDK's custom popup permission.
|
Pop-up launched. User denied permission.
|
|
Error
|
Something went wrong with error --- <error>
|
Updated 7 months ago