Notification Runtime Permission (Android)
Android 13 (API level 33) and higher supports runtime permission for sending non-exempt (including Foreground Services (FGS)) notifications from an app. We recommend targeting Android 13 or higher as soon as possible to benefit from this feature's additional control and flexibility. Click here to learn more about push prompt notification permission.
Import Package
import { PushPromptInfo } from 'react-native-notifyvisitors';
import 'package:flutter_notifyvisitors/PushPromptInfo.dart';
declare var NVPopupDesign: any;
Add the undermentioned code to your class
let design = new PushPromptInfo();
design.setTitle = "Test Title";
design.setTitleTextColor = "#000000";
design.setDescription = "Enable Push Notifications on Your Device !!";
design.setDescriptionTextColor = "#000000";
design.setBackgroundColor = "#EBEDEF";
design.setButtonOneBorderColor = "#6db76c";
design.setButtonOneBackgroundColor = "#26a524";
design.setButtonOneBorderRadius = 0;
design.setButtonOneText = "Allow";
design.setButtonOneTextColor = "#FFFFFF";
design.setButtonTwoText = "Cancel";
design.setButtonTwoTextColor = "#FFFFFF";
design.setButtonTwoBackgroundColor = "#FF0000";
design.setButtonTwoBorderColor = "#6db76c";
design.setButtonTwoBorderRadius = 0;
design.setNumberOfSessions = 3;
design.setResumeInDays = 1;
design.setNumberOfTimesPerSession = 6;
NotifyVisitors.pushPermissionPrompt(design, function (response) {
//console.log(response);
});
PushPromptInfo design = new PushPromptInfo();
design.title = "Test Title";
design.titleTextColor = "#000000";
design.description = "Enable Push Notifications on Your Device !!";
design.descriptionTextColor = "#000000";
design.backgroundColor = "#EBEDEF";
design.buttonOneBorderColor = "#6db76c";
design.buttonOneBackgroundColor = "#26a524";
design.buttonOneBorderRadius = 0;
design.buttonOneText = "Allow";
design.buttonOneTextColor = "#FFFFFF";
design.buttonTwoText = "Cancel";
design.buttonTwoTextColor = "#FFFFFF";
design.buttonTwoBackgroundColor = "#FF0000";
design.buttonTwoBorderColor = "#6db76c";
design.buttonTwoBorderRadius = "0";
design.numberOfSessions = "3";
design.setResumeInDays = "1";
design.setNumberOfTimesPerSession = "6";
Notifyvisitors.shared.pushPermissionPrompt(design, (response) {
//do your task
});
let design = new NVPopupDesign();
design.setTitle = "Test Title";
design.setTitleTextColor = "#000000";
design.setDescription = "Enable Push Notifications on Your Device !!";
design.setDescriptionTextColor = "#000000";
design.setBackgroundColor = "#EBEDEF";
design.setButtonOneBorderColor = "#6db76c";
design.setButtonOneBackgroundColor = "#26a524";
design.setButtonOneBorderRadius = 0;
design.setButtonOneText = "Allow";
design.setButtonOneTextColor = "#FFFFFF";
design.setButtonTwoText = "Cancel";
design.setButtonTwoTextColor = "#FFFFFF";
design.setButtonTwoBackgroundColor = "#FF0000";
design.setButtonTwoBorderColor = "#6db76c";
design.setButtonTwoBorderRadius = 0;
design.setNumberOfSessions = 3;
design.setResumeInDays = 1;
design.setNumberOfTimesPerSession = 6;
NotifyVisitors.activatePushPermissionPopup(design, function (show) {
alert("show response : " + show);
});
Notice that there are two parameters in the function above namely numberOfSessions and resumeInDays. These two parameters will handle the flow of SDK’s push prompt permission (not system’s permission dialog).
numberOfSessions → This parameter takes integer as input. In NV SDK, new or next session is created either on first app launch or after 30 minutes of user’s inactivity. So, if you set 3, then prompt will be displayed for next 3 sessions including current session in case user denies the prompt in every attempt.
resumeInDays → This parameter takes integer as input. When numberOfSessions set by you is completed then resumeInDays parameter will work. This is used to control the time period in days after which you intend to show the prompt again to the user. So, if you set 10 then prompt will remain hidden for 10 days and from the 11th day onwards it will get displayed according to the condition set in numberOfSessions parameter.
Callback responses
STATUS
|
MESSAGE
|
Success |
Popup launched. User granted permission. |
Success |
Push permission is already active on this device. |
Fail |
According to the resumeInDays set by the user, next popup will be shown after <day> day(s). |
Fail |
Session count for popup set by the user has been exceeded. Next popup will resume after resumeInDays condition falls false. |
Fail
|
Session count for popup set by the user has been exceeded. Next popup will resume after resumeInDays condition falls false.
|
Fail
|
Popup launched. User denied SDK's custom popup permission.
|
Fail
|
Popup launched. User denied permission.
|
Fail
|
Something went wrong with error --> <error>
|
Error
|
Failed with error :: <error>
|
Error
|
Something went wrong while launching popup with error :: <error>
|
Error
|
Something went wrong with error -----> <error>
|
Output with callback
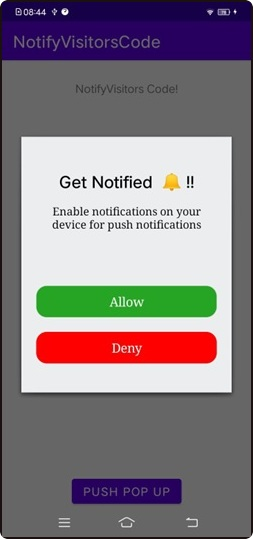
{
"status":"success",
"message":"Popup launched. User granted permission."
}
Updated 8 months ago